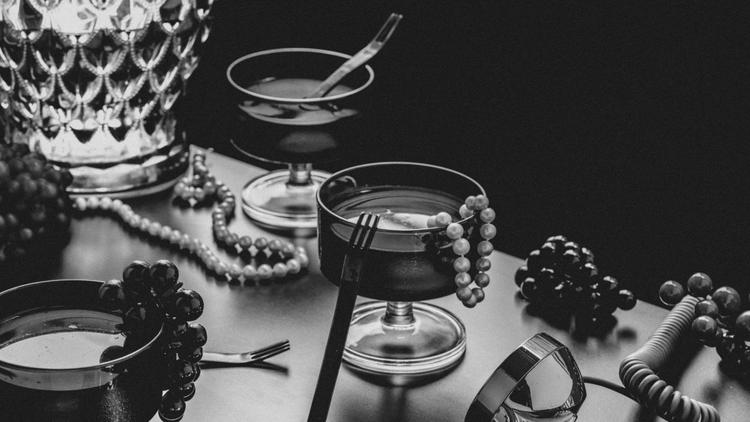
Getting dynamic data from Contentful using GatsbyJS
Get dynamic data in Gatsby with Apollo - the solution to useStaticQuery for build-time data retrieval.
Loading dynamic content with Apollo in Gatsby is necessary because Gatsby is a static site generator that generates static HTML, CSS, and JavaScript files at build time. This means that all the data needed to render your site must be available at build time.
However, in many cases, you may need to load data dynamically from a remote data source, such as a GraphQL API, in order to provide up-to-date content to your users. This is where Apollo comes in - it provides a way to fetch and manage data from a GraphQL API, which can be used to load dynamic content in Gatsby.
To load dynamic content with Apollo in Gatsby, you can use the gatsby-plugin-apollo
plugin. This plugin allows you to connect your Gatsby site to an Apollo client, which you can use to fetch and manage data from a GraphQL API.
Here are the general steps you would take to load dynamic content with Apollo in Gatsby:
Install the gatsby-plugin-apollo
plugin: Use npm or yarn to install the gatsby-plugin-apollo
plugin.
npm install gatsby-plugin-apollo @apollo/client graphql
Define your Apollo client: In your gatsby-config.js
file, define your Apollo client and any necessary configuration options. For example:
module.exports = {
plugins: [
{
resolve: 'gatsby-plugin-apollo',
options: {
uri: 'https://example.com/graphql',
},
},
],
};
This configuration sets the URI of your GraphQL API.
Write a GraphQL query: In your Gatsby page or component, write a GraphQL query using the useQuery
hook provided by Apollo. For example:
import { useQuery, gql } from '@apollo/client';
const MY_QUERY = gql`
query MyQuery {
someData {
id
name
description
}
}
`;
function MyComponent() {
const { loading, error, data } = useQuery(MY_QUERY);
if (loading) {
return <p>Loading...</p>;
}
if (error) {
return <p>Error: {error.message}</p>;
}
return (
<div>
<h1>{data.someData.name}</h1>
<p>{data.someData.description}</p>
</div>
);
}
This query fetches some data from your GraphQL API and uses it to render your component.
By using the gatsby-plugin-apollo
plugin and the Apollo client, you can fetch data from your GraphQL API at runtime, and use it to update your Gatsby pages and components. This enables you to create dynamic, data-driven sites with Gatsby, while still taking advantage of its benefits as a static site generator.